basics (Spring 2015)
Contents
iminfo
info = imfinfo(filename) returns a structure whose fields contain information about an image in a graphics file. filename is a string that specifies the name of the graphics file
clear close all filename = '2013_0115_163116AA.JPG'; info = imfinfo(filename); disp(info)
Filename: 'C:\Users\jloomis1\Google Drive\ece564\2015\basics20...' FileModDate: '16-Jan-2013 22:31:05' FileSize: 607096 Format: 'jpg' FormatVersion: '' Width: 2048 Height: 1536 BitDepth: 24 ColorType: 'truecolor' FormatSignature: '' NumberOfSamples: 3 CodingMethod: 'Huffman' CodingProcess: 'Sequential' Comment: {} Make: 'FUJIFILM' Model: 'FinePix6900ZOOM' Orientation: 1 XResolution: 72 YResolution: 72 ResolutionUnit: 'Inch' Software: 'Digital Camera FinePix6900ZOOM Ver1.00' DateTime: '2013:01:15 16:31:16' YCbCrPositioning: 'Co-sited' Copyright: ' ' DigitalCamera: [1x1 struct] ExifThumbnail: [1x1 struct]
additional fields
which leads us to enter:
disp('info.DigitalCamera'); disp(info.DigitalCamera); if isfield(info,'GPSInfo') disp('info.GPSInfo'); disp(info.GPSInfo); end disp('info.ExifThumbnail'); disp(info.ExifThumbnail);
info.DigitalCamera FNumber: 2.8000 ExposureProgram: 'Normal program' ISOSpeedRatings: 100 ExifVersion: [48 50 49 48] DateTimeOriginal: '2013:01:15 16:31:16' DateTimeDigitized: '2013:01:15 16:31:16' ComponentsConfiguration: 'YCbCr' CompressedBitsPerPixel: 1.5000 ShutterSpeedValue: 6 ApertureValue: 3 BrightnessValue: 3.1100 ExposureBiasValue: 0 MaxApertureValue: 3 MeteringMode: 'Pattern' Flash: 'Flash fired, no strobe return detection fun...' FocalLength: 9.7000 MakerNote: [1x238 double] FlashpixVersion: [48 49 48 48] ColorSpace: 'sRGB' CPixelXDimension: 2048 CPixelYDimension: 1536 InteroperabilityIFD: [1x1 struct] FocalPlaneXResolution: 2678 FocalPlaneYResolution: 2674 FocalPlaneResolutionUnit: 3 SensingMethod: 'One-chip color area sensor' FileSource: 'DSC' SceneType: 'A directly photographed image' info.ExifThumbnail Compression: 'OJPEG' Orientation: 1 XResolution: 72 YResolution: 72 ResolutionUnit: 'Inch' JPEGInterchangeFormat: 1102 JPEGInterchangeFormatLength: 9225 YCbCrPositioning: 'Co-sited'
imread
A = imread(filename) reads a grayscale or color image from the file specified by the string filename.
rgb = imread(filename);
whos rgb
Name Size Bytes Class Attributes rgb 1536x2048x3 9437184 uint8
imshow
imshow(RGB) displays the truecolor image RGB.
small = imresize(rgb,0.3); imshow(small);
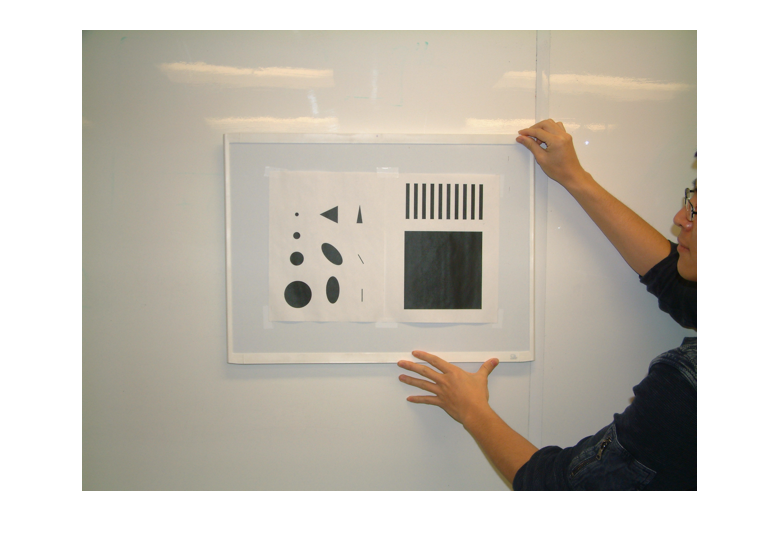
imcrop
I = imcrop creates an interactive Crop Image tool associated with the image displayed in the current figure, called the target image. The Crop Image tool is a moveable, resizable rectangle that you can position interactively using the mouse.
rect = [637.5 484.5 732 470]; imshow(rgb); if exist('rect','var') out = imcrop(rgb,rect); hold on x1 = rect(1); y1 = rect(2); x2 = x1+rect(3); y2 = y1+rect(4); plot([x1 x2 x2 x1 x1],[y1 y1 y2 y2 y1],'b-','Linewidth',2); hold off else [out rect] = imcrop; rect end
Warning: Image is too big to fit on screen; displaying at 33%
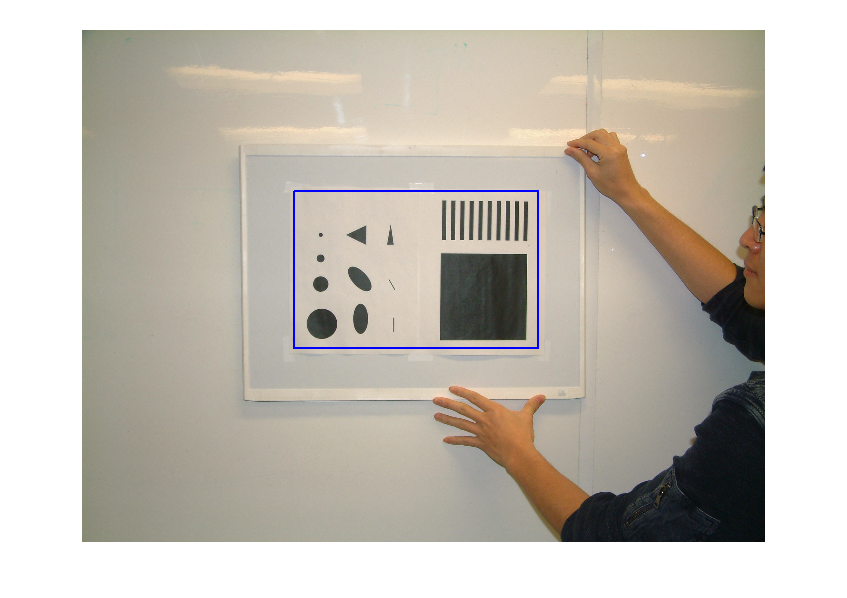
imwrite
imwrite(A,filename) writes the image A to the file specified by filename in the format specified the file extension.
imwrite(out,'cropped_target.jpg');
imshow(out);
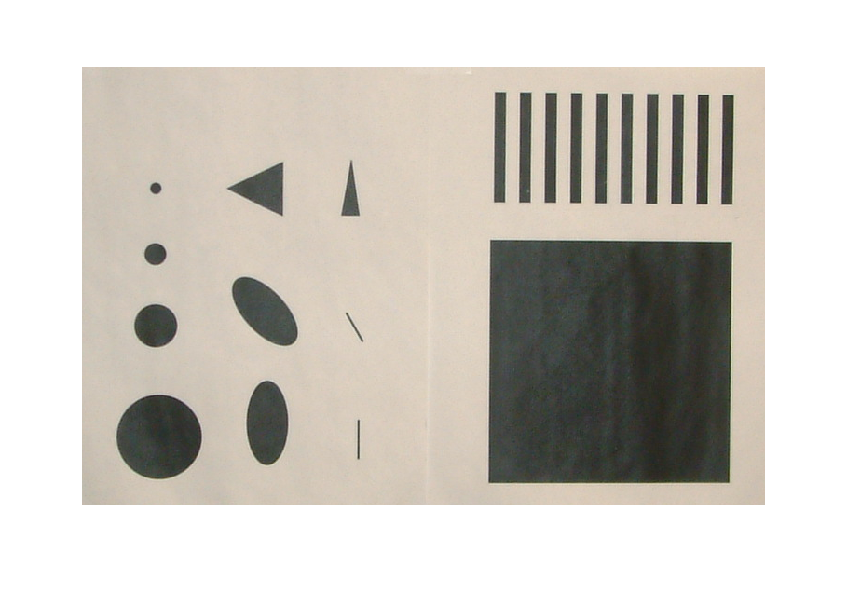
rgb2gray
I = rgb2gray(RGB) converts the truecolor image RGB to a grayscale intensity image I. rgb2gray converts RGB images to grayscale by eliminating the hue and saturation information while retaining the luminance.
g = rgb2gray(out); imshow(g);
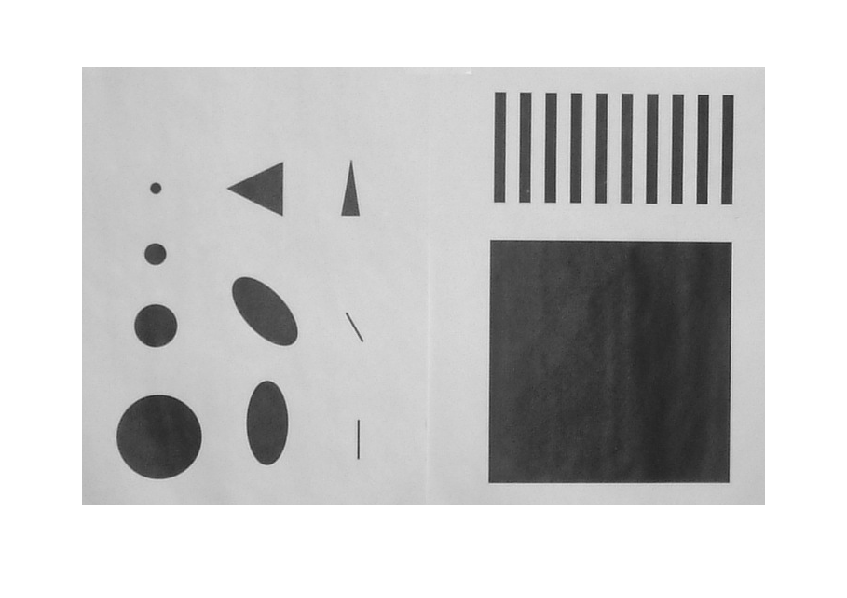
imhist
imhist(I) displays a histogram for the image I above a grayscale colorbar. The number of bins in the histogram is specified by the image type. If I is a grayscale image, imhist uses a default value of 256 bins.
imhist(im2double(g));
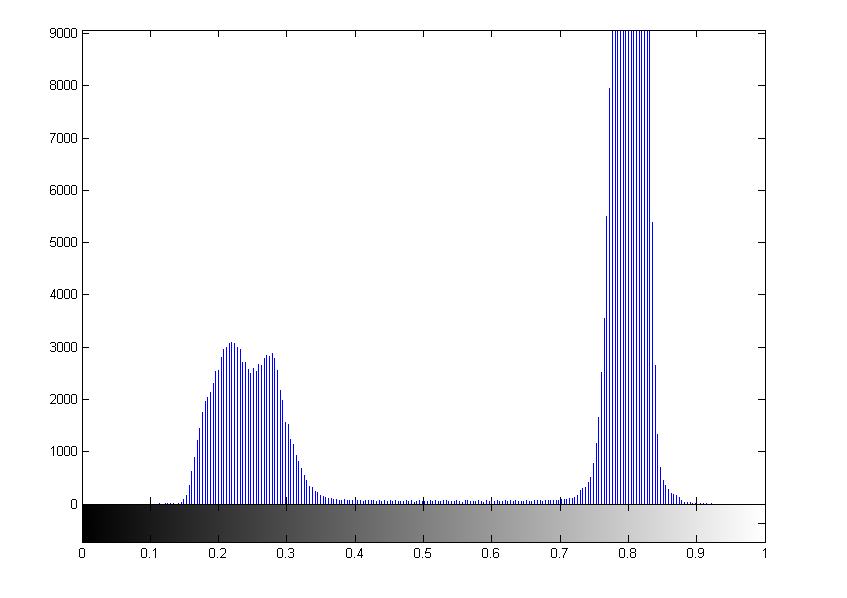
graythresh
level = graythresh(I) computes a global threshold (level) that can be used to convert an intensity image to a binary image with im2bw. level is a normalized intensity value that lies in the range [0, 1].
%The graythresh function uses Otsu's method, which chooses the threshold % to minimize the intraclass variance of the black and white pixels. level = graythresh(g); fprintf('threshold %g\n',level); hold on plot([level level],[0 1000],'k-','Linewidth',2); hold off
threshold 0.521569
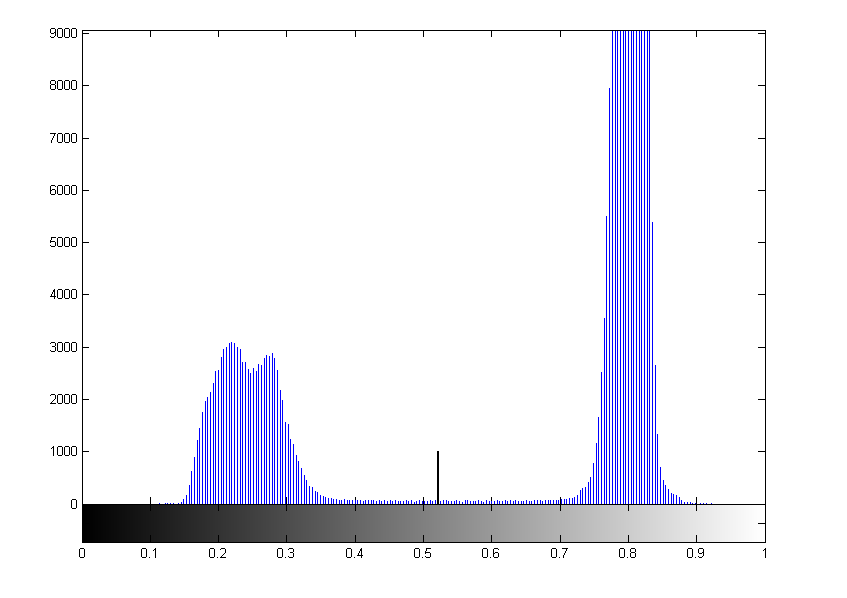
im2bw
BW = im2bw(g,level); imshow(BW);
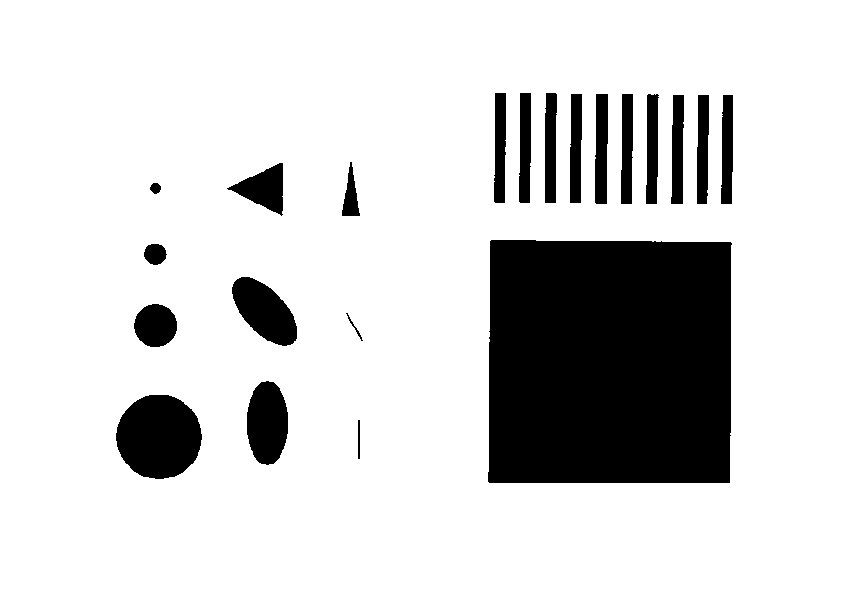
bwconncomp
CC = bwconncomp(BW) returns the connected components CC found in BW. The binary image BW can have any dimension. CC is a structure with four fields.
CC = bwconncomp(~BW); disp(CC)
Connectivity: 8 ImageSize: [471 733] NumObjects: 21 PixelIdxList: {1x21 cell}
labelmatrix
L = labelmatrix(CC) creates a label matrix from the connected components structure CC returned by bwconncomp. The size of L is CC.ImageSize. The elements of L are integer values greater than or equal to 0.
L = labelmatrix(CC);
whos L
Name Size Bytes Class Attributes L 471x733 345243 uint8
label2rgb
RGB = label2rgb(L) converts a label matrix into an RGB color image for the purpose of visualizing the labeled regions.
RGB = label2rgb(L); imshow(RGB);
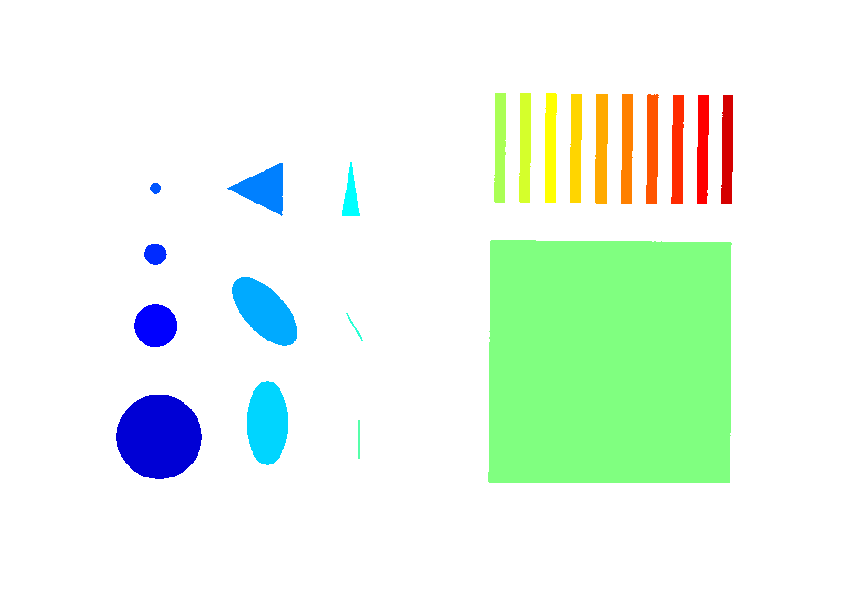
histogram (integer based)
imhist(g); hold on plot([100 100],[0 1000],'k-','Linewidth',2); plot([170 170],[0 1000],'k-','Linewidth',2); hold off
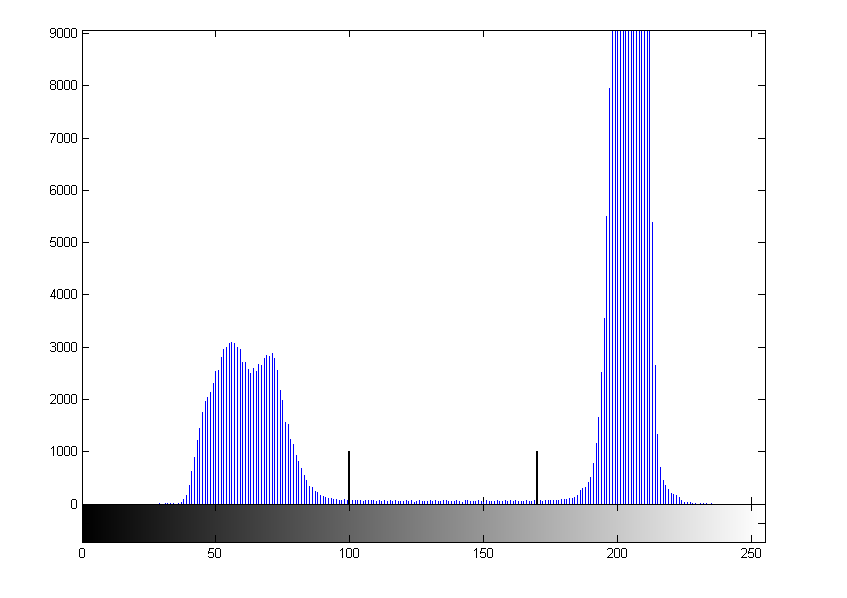
simple mask operations
show "inbetween" values;
msk1 = g>100; msk2 = g<170; imshow(msk1&msk2);
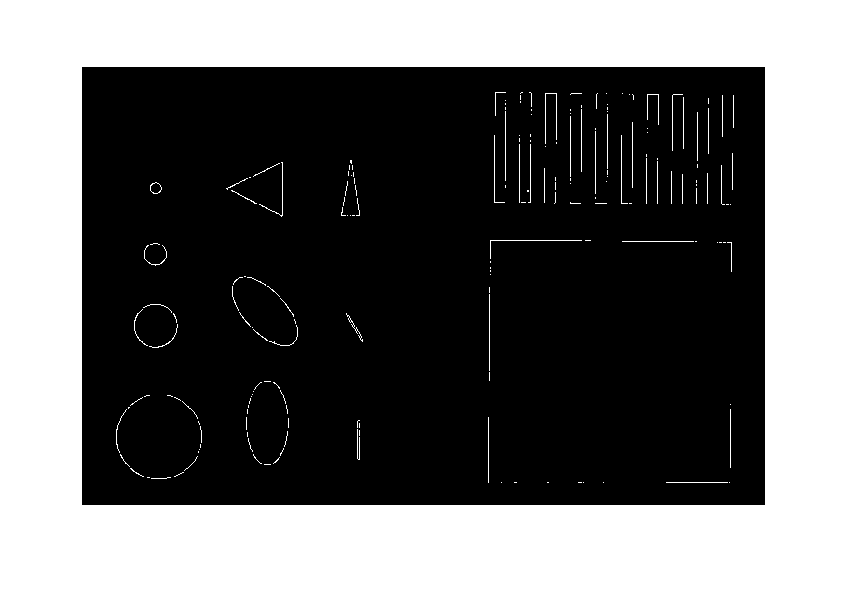